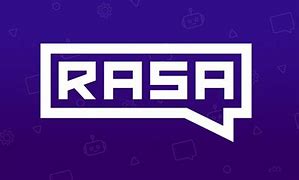
Rasa 4D
Session configuration#
A conversation session represents the dialogue between the assistant and the user. Conversation sessions can begin in three ways:
the user begins the conversation with the assistant,
the user sends their first message after a configurable period of inactivity, or
a manual session start is triggered with the /session_start intent message.
You can define the period of inactivity after which a new conversation session is triggered in the domain under the session_config key.
Available parameters are:
The default session configuration looks as follows:
session_expiration_time: 60 # value in minutes, 0 means infinitely long
carry_over_slots_to_new_session: true # set to false to forget slots between sessions
This means that if a user sends their first message after 60 minutes of inactivity, a new conversation session is triggered, and that any existing slots are carried over into the new session. Setting the value of session_expiration_time to 0 means that sessions will not end (note that the action_session_start action will still be triggered at the very beginning of conversations).
A session start triggers the default action action_session_start. Its default implementation moves all existing slots into the new session. Note that all conversations begin with an action_session_start. Overriding this action could for instance be used to initialize the tracker with slots from an external API call, or to start the conversation with a bot message. The docs on Customizing the session start action shows you how to do that.
Custom Slot Mappings#
If none of the predefined Slot Mappings fit your use
case, you can use the
Custom Action validate_
If you're using the Rasa SDK we recommend you to extend the provided FormValidationAction. When using the FormValidationAction, three steps are required to extract customs slots:
The following example shows the implementation of a form which extracts a slot outdoor_seating in a custom way, in addition to the slots which use predefined mappings. The method extract_outdoor_seating sets the slot outdoor_seating based on whether the keyword outdoor was present in the last user message.
from typing import Dict, Text, List, Optional, Any
from rasa_sdk import Tracker
from rasa_sdk.executor import CollectingDispatcher
from rasa_sdk.forms import FormValidationAction
class ValidateRestaurantForm(FormValidationAction):
def name(self) -> Text:
return "validate_restaurant_form"
async def required_slots(
slots_mapped_in_domain: List[Text],
dispatcher: "CollectingDispatcher",
domain: "DomainDict",
) -> Optional[List[Text]]:
required_slots = slots_mapped_in_domain + ["outdoor_seating"]
return required_slots
async def extract_outdoor_seating(
self, dispatcher: CollectingDispatcher, tracker: Tracker, domain: Dict
) -> Dict[Text, Any]:
text_of_last_user_message = tracker.latest_message.get("text")
sit_outside = "outdoor" in text_of_last_user_message
return {"outdoor_seating": sit_outside}
By default the FormValidationAction will automatically set the requested_slot to the first slot specified in required_slots which is not filled.
Using a Custom Action to Ask For the Next Slot#
As soon as the form determines which slot has to be filled next by the user, it will
execute the action utter_ask_
from typing import Dict, Text, List
from rasa_sdk import Tracker
from rasa_sdk.events import EventType
from rasa_sdk.executor import CollectingDispatcher
from rasa_sdk import Action
class AskForSlotAction(Action):
def name(self) -> Text:
return "action_ask_cuisine"
self, dispatcher: CollectingDispatcher, tracker: Tracker, domain: Dict
) -> List[EventType]:
dispatcher.utter_message(text="What cuisine?")
Here is a full example of a domain, taken from the concertbot example:
influence_conversation: false
influence_conversation: false
influence_conversation: true
- text: "Sorry, I didn't get that, can you rephrase?"
- text: "You're very welcome."
- text: "I am a bot, powered by Rasa."
- text: "I can help you find concerts and venues. Do you like music?"
- text: "Awesome! You can ask me things like \"Find me some concerts\" or \"What's a good venue\""
- action_search_concerts
- action_search_venues
- action_show_concert_reviews
- action_show_venue_reviews
- action_set_music_preference
session_expiration_time: 60 # value in minutes
carry_over_slots_to_new_session: true
Validating Form Input#
After extracting a slot value from user input, you can validate the extracted slots. By default Rasa Open Source only validates if any slot was filled after requesting a slot.
Forms no longer raise ActionExecutionRejection if nothing is extracted from the user’s utterance for any of the required slots.
You can implement a Custom Action validate_
- validate_restaurant_form
When the form is executed it will run your custom action.
This custom action can extend FormValidationAction class to simplify
the process of validating extracted slots. In this case, you need to write functions
named validate_
The following example shows the implementation of a custom action which validates that the slot named cuisine is valid.
from typing import Text, List, Any, Dict
from rasa_sdk import Tracker, FormValidationAction
from rasa_sdk.executor import CollectingDispatcher
from rasa_sdk.types import DomainDict
class ValidateRestaurantForm(FormValidationAction):
def name(self) -> Text:
return "validate_restaurant_form"
def cuisine_db() -> List[Text]:
"""Database of supported cuisines"""
return ["caribbean", "chinese", "french"]
def validate_cuisine(
dispatcher: CollectingDispatcher,
) -> Dict[Text, Any]:
"""Validate cuisine value."""
if slot_value.lower() in self.cuisine_db():
return {"cuisine": slot_value}
return {"cuisine": None}
You can also extend the Action class and retrieve extracted slots with tracker.slots_to_validate to fully customize the validation process.
The requested_slot slot#
The slot requested_slot is automatically added to the domain as a slot of type text. The value of the requested_slot will be ignored during conversations. If you want to change this behavior, you need to add the requested_slot to your domain file as a categorical slot with influence_conversation set to true. You might want to do this if you want to handle your unhappy paths differently, depending on what slot is currently being asked from the user. For example, if your users respond to one of the bot's questions with another question, like why do you need to know that? The response to this explain intent depends on where we are in the story. In the restaurant case, your stories would look something like this:
- story: explain cuisine slot
- intent: request_restaurant
- action: restaurant_form
- active_loop: restaurant
- requested_slot: cuisine
- action: utter_explain_cuisine
- action: restaurant_form
- story: explain num_people slot
- intent: request_restaurant
- action: restaurant_form
- active_loop: restaurant
- requested_slot: cuisine
- requested_slot: num_people
- action: utter_explain_num_people
- action: restaurant_form
Again, it is strongly recommended that you use interactive learning to build these stories.
Custom Slot Mappings#
You can define custom slot mappings using slot validation actions when none of the predefined mappings fit your use case. You must define this slot mapping to be of type custom, for example:
action: action_calculate_day_of_week
You can also use the custom slot mapping to list slots that will be filled by arbitrary custom actions in the course of a conversation, by listing the type and no specific action. For example:
This slot will not be updated on every user turn, but only once a custom action that returns a SlotSet event for it is predicted.
You can provide an initial value for a slot in your domain file:
Responses are actions that send a message to a user without running any custom code or returning events. These responses can be defined directly in the domain file under the responses key and can include rich content such as buttons and attachments. For more information on responses and how to define them, see Responses.
Forms are a special type of action meant to help your assistant collect information from a user. Define forms under the forms key in your domain file. For more information on form and how to define them, see Forms.
Actions are the things your bot can actually do. For example, an action could:
All custom actions should be listed in your domain, except responses which need not be listed under actions: as they are already listed under responses:.
Custom Output Payloads#
You can send any arbitrary output to the output channel using the custom key. The output channel receives the object stored under the custom key as a JSON payload.
Here's an example of how to send a date picker to the Slack Output Channel:
text: "Make a bet on when the world will end:"
initial_date: '2019-05-21'
Using Responses in Conversations#
Ignoring Entities for Certain Intents#
To ignore all entities for certain intents, you can add the use_entities: [] parameter to the intent in your domain file like this:
To ignore some entities or explicitly take only certain entities into account you can use this syntax:
You can only use_entities or ignore_entities for any single intent.
Excluded entities for those intents will be unfeaturized and therefore will not impact the next action predictions. This is useful when you have an intent where you don't care about the entities being picked up.
If you list your intents without a use_entities or ignore_entities parameter, the entities will be featurized as normal.
It is also possible to ignore an entity for all intents by setting the influence_conversation flag to false for the entity itself. See the entities section for details.
Excluded entities for intents will be unfeaturized and therefore will not impact the next action predictions. This is useful when you have an intent where you don't care about the entities being picked up.
If you list your intents without this parameter, and without setting influence_conversation to false for any entities, all entities will be featurized as normal.
If you want these entities not to influence action prediction via slots either, set the influence_conversation: false parameter for slots with the same name.
As of 3.1, you can use the influence_conversation flag under entities. The flag can be set to false to declare that an entity should not be featurized for any intents. It is a shorthand syntax for adding an entity to the ignore_entities list of every intent in the domain. The flag is optional and default behaviour remains unchanged.
The entities section lists all entities that can be extracted by any entity extractor in your NLU pipeline.
- PERSON # entity extracted by SpacyEntityExtractor
- time # entity extracted by DucklingEntityExtractor
- membership_type # custom entity extracted by DIETClassifier
- priority # custom entity extracted by DIETClassifier
When using multiple domain files, entities can be specified in any domain file, and can be used or ignored by any intent in any domain file.
If you are using the feature Entity Roles and Groups you also need to list the roles and groups of an entity in this section.
- city: # custom entity extracted by DIETClassifier
- topping: # custom entity extracted by DIETClassifier
- size: # custom entity extracted by DIETClassifier
By default, entities influence action prediction. To prevent extracted entities from influencing the conversation for specific intents you can ignore entities for certain intents. To ignore an entity for all intents, without having to list it under the ignore_entities flag of each intent, you can set the flag influence_conversation to false under the entity:
influence_conversation: false
This syntax has the same effect as adding the entity to the ignore_entities list for every intent in the domain.
Explicitly setting influence_conversation: true does not change any behaviour. This is the default setting.
Slots are your bot's memory. They act as a key-value store which can be used to store information the user provided (e.g their home city) as well as information gathered about the outside world (e.g. the result of a database query).
Slots are defined in the slots section of your domain with their name, type and if and how they should influence the assistant's behavior. The following example defines a slot with name "slot_name", type text and predefined slot mapping from_entity.
Select which actions should receive domain#
You can control if an action should receive a domain or not.
To do this you must first enable selective domain in you endpoint configuration for action_endpoint in endpoints.yml.
url: "http://localhost:5055/webhook" # URL to your action server
enable_selective_domain: true
After selective domain for custom actions is enabled, domain will be sent only to those custom actions which have specifically stated that they need it. Custom actions inheriting from rasa-sdk FormValidationAction parent class are an exception to this rule as they will always have the domain sent to them. To specify if an action needs the domain add {send_domain: true} to custom action in the list of actions in domain.yml:
- action_hello_world: {send_domain: True} # will receive domain
- action_calculate_mass_of_sun # will not receive domain
- validate_my_form # will receive domain